Object Orientation in PHP
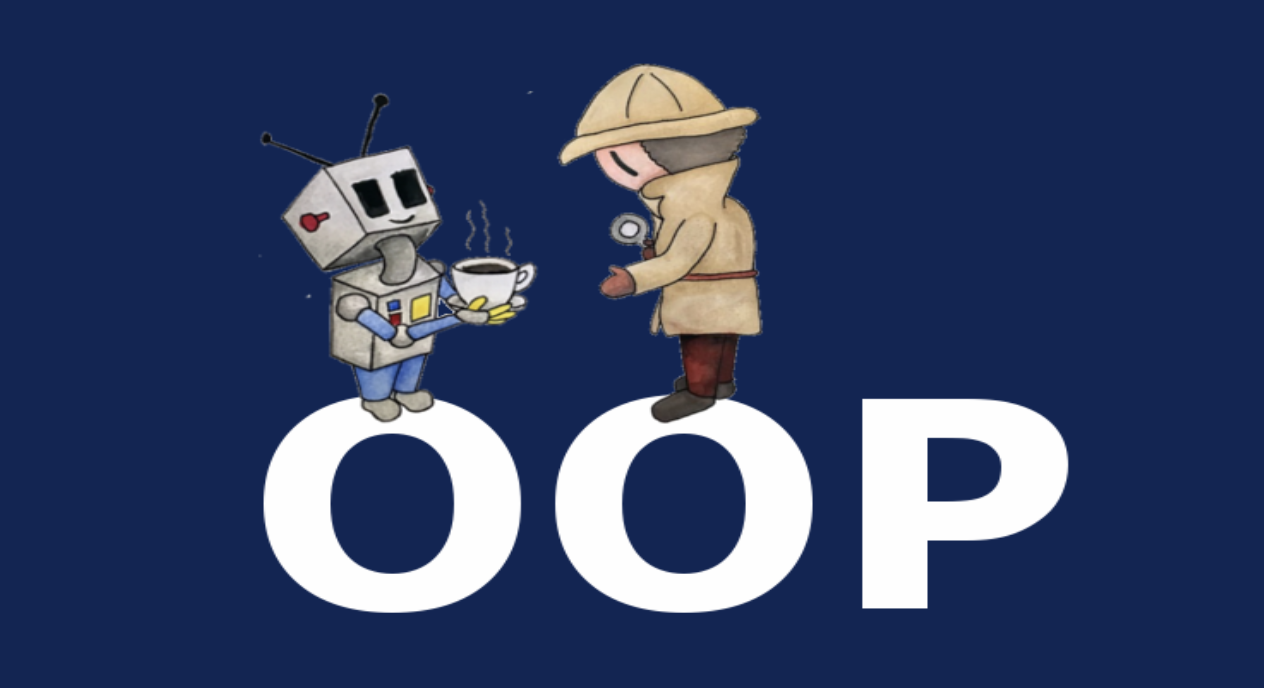
Object-Oriented Programming, also known as OOP, is a method of object-based programming. This method allows programmers to write clean, higher-maintenance, and more accessible debugging code. This programming style is very similar to real-world concepts; The brain, for example, is object-oriented in that it receives information from the environment and responds appropriately after processing it. In the following, we will get acquainted with the concepts of object orientation in PHP and some examples; Stay with us.
Learn more: What is Object Oriented Programming?
The benefits of object orientation in PHP
Object-oriented programming has many advantages, including splitting code into smaller sections, organizing and managing code, avoiding duplication, simply applying the previous code, more program security, easier and faster debugging, and so on.
Object-Oriented Programming Concepts in PHP
here we discuss the Object-Oriented Programming Concepts in PHP, and in the next step will explain them with examples.
Class:
A type of data defined by a programmer that contains local functions and data. You can consider a class as a model for building many instances of the same type.
Object:
An object is a single instance of a data structure defined by a class. You define a class once and then assign your favourite objects to it. Objects are also known as examples.
Member Variable:
Variables that are defined within a class. This data are invisible outside the class and can be accessed through member functions. When an object is created, these variables are called object attributes.
Member function:
The function is defined within a class and is used to access object data.
Inheritance:
When a class is defined by inheriting the existing function of a parent class, it is called inheritance. A child class inherits all or some of the functions and variables of the parent class and can also have its own unique functions and variables.
Parent class:
The class from which another class inherits is called the parent class. This class is also called the base or upper class.
Child Class:
A class that inherits from another class is called a child class. This class is also called a subclass or derived class.
Polymorphism:
An object-oriented concept in which one function can be used for different purposes. For example, the function name remains the same but takes different arguments and can do various things.
Overloading:
A type of polymorphism in which some or all operators have different implementations depending on the type of their arguments. Similar functions can be overloaded with different performances.
Data Abstraction:
Abstraction helps the programmer to hide the details of the program implementation from the user. It causes only operations that are related to other objects in the program to be displayed. Abstraction methods may or may not have a body.
Interface:
The interface is the same as the abstraction, except that its methods have no body, and the class that implements the interface is required to rewrite all parent class methods. The similarity between interfaces and abstraction is that no object can be created from either.
Encapsulation:
A concept in which we combine all the data and functions of a member to form a new object. In encapsulation, access to objects is restricted by leveling.
Constructor:
A special type of function that is called automatically whenever an object consists of a class.
Destructor:
A particular type of function that is called automatically whenever an object is removed or removed from the domain.
Define class in PHP
The class is defined using the class keyword, followed by the class name and a pair of parentheses.
The class definition in PHP is as follows:
In the below example we have made a class called smartphone with two properties ($brand and $size) and two methods set_brand() and get_brand () to set and get the $brand property:
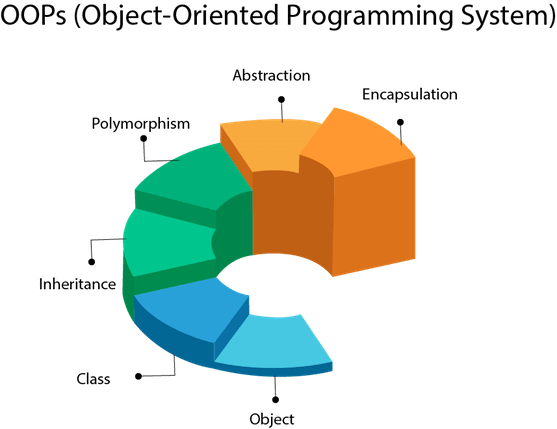
Define Object in PHP
You can make various objects from one class. Each object has all the properties and methods on the class, but it has different values of properties. You can determine objects in a class by the word ”new”.
In the below example, $Apple and $Samsung are objects.
Call Member Functions
After creating the objects, you will be able to call the member functions associated with that object. A member function can only process the member variable from the associated object.
Consider the following example:
Then we create three independent objects of book groups:
The following code shows how to set the title and price of these three books by calling member functions:
We now call other member functions to get the values set in the example above.
Constructor Functions in PHP
In PHP, the constructor method specifies a class named _construct. Most programmers use this method for operations such as initializing object properties. If a constructor method is defined in a class, when it creates an object in the class, its constructor method will be called automatically. A constructor method can also receive arguments and perform initialization operations based on them.
The following example creates a constructor for the Books class and values the price and title of the book when creating the object.
Now we do not need to call the set function to set the price and title. We can initialize these two member variables only when the object is created. See the following example:
Public and Private Access Levels
Each member of a class is defined in two levels of public access or private. These access levels refer to the possibility / impossibility of accessing these elements from outside the classroom. A public member can be accessed anywhere in a PHP application. Conversely, a private or confidential member is only accessible to class members and cannot be accessed from outside the classroom.
In object-oriented programming, attributes (variables) are generally defined privately and methods (functions) are defined publicly. The term encapsulation is also used for this purpose. This means that the data of a class (attributes) must be hidden from other parts of the program (outside the class). Instead, if they need to be accessed, this is done indirectly through class member functions (methods).
Private Member Function
Although we have already stated that in object-oriented programming in PHP, class methods are introduced publicly. However, sometimes we have to define methods privately. This mode is used when the function does not need to be called from outside the class and is used only as an auxiliary function inside the class. Such a function is called a private member function.
In the code snippet above, we define the standard_time () method as a private member function (lines 21 to 38). The purpose of this function is to print the desired time in a 12-hour format (with AM and PM symbols). Outside of the class, we have set and printed the time1 object twice. Note the manual call to the constructor function on line 47.
The output of the above code snippet is as follows:
Static Class Member
Sometimes we want to access class members without any object in the class. In this case, we define that member as a static member. Until now, access to class members (specifically methods) was done through an existing object. A static member is a unique and common member that can be accessed without dependence on the object.
In the above code snippet, both the data members of the class (attributes) and the function members of the class (methods) are defined as static members. Note how to access static class attributes within the print_time () method (line 11). How to access the static method is also shown in line 15. Since there is no object, we have accessed the print_time () function using the class name and the delimiter ::.
The output of the above code snippet is the same as before.
Leave a Comment