Singleton Design Pattern With a Practical Example
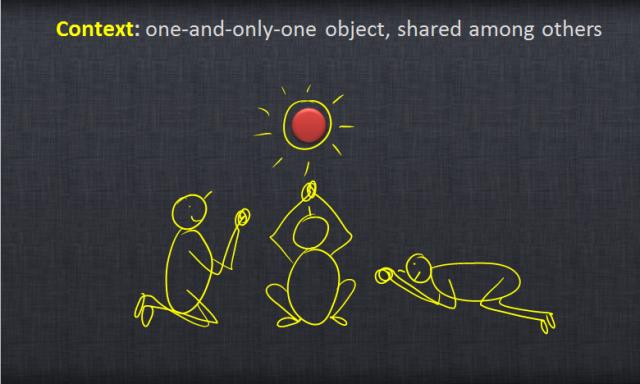
In the article ‘Design Patterns, Definitions, History, and Categories,’ we mentioned experienced programmers had created design patterns to solve common problems in object-oriented programming. These types of issues are independent of programming languages. One of the most widely used design patterns is the ‘Singleton design pattern,’ which we will get acquainted with within this article.

What is Singleton?
Singleton is a Creational design pattern that assures you only one instance is made up of a class. Whenever you need to use this class, Singleton provides a global access point to this class’s only object and does not allow you to create a new one.
What problems does Singleton‘s design pattern solve?
The singleton pattern violates Single Responsibility. This template can solve two problems at the same time:
- Make sure that only one object is created in a class.
- It provides global access to this item.
But you may be wondering why there should not be more than one instance of a class?
Each of these constructed objects takes up a portion of the resources; thus, the most common reason for the restriction, is to control access to some shared resources. Imagine that you have created an object of a class, but you decide to make another one after a while. In this case, if you do not use the Singleton design pattern, instead of using the object you have already created, you will make a new instance, which is costly and non-optimal in terms of resource consumption.
It is interesting to note that even clients may not realize that they have been using one object all along.

What if we do not use the Singleton design pattern?
TWO CAPTAINS WILL SHRINK THE SHIP.
Imagine programming as piloting a ship. The “class” is the ship’s captain who determines the shipping methods (class methods). We create an instance of the class and name it ‘Captain.’
If we do not use Singleton’s design pattern here, it is possible to re-create a new class instance. Thus, the number of captains will increase. Making this mistake, in addition to consuming more space and food (system resources), the ship is more likely to sink. (Software will have many problems.)
Singleton design pattern in programming
Usually, in software, the task of communicating with the database is assigned to an object. It is best to use the Singleton design pattern to make sure no other class instances are created.
With this method, we don’t need to create a new object for each connection to the database, and we will use the same one forever just by creating an instance of DbConnection class. Accordingly, once we use the “new” keyword in coding, then we only use the “getInstance” (String algorithm) function of the Singleton design pattern.
The pattern is responsible for providing access to the only instance of the DBConnection class. It is necessary not to build more than one object from this class, as in addition to consuming more memory, it may also lead to software problems.

How to implement the Singleton design pattern
In this part of the article, we first teach you how to implement the Singleton design pattern and then implement it for the DbConnection class using the PHP programming language.
In general, a class must have one function to create an object of that class if it was not previously made. To ensure that we do not create another instance of this class, we must define the class constructor access as Private.
Singleton implementation steps are as follow:
- Add a Private static variable to the class to store the Singleton instance in it.
- Write a Static function of Public type to get a Singleton instance. (this function will have no input).
- Implement “lazy initialization” inside the Static function. In this step, a new object must be created in the first call and puts its value in the Static variable. The method should always return that instance on all subsequent calls.
- Privatize the constructor for the class and the class from which it is inherited.
- By doing this, we will no longer be able to create an object outside of the class; this will only be possible in the same class.
- Finally, in coding, we should use the Static function we wrote instead of directly calling the Constructor Singleton (Direct calls).
<?php
/*
* DBConnection class – only one connection allowed
*/
class DBConnection {
private $connection;
private static $instance; //The static variable for store Singleton’s instance
private $host = “HOSTt”;
private $username = “USERNAME”;
private $password = “PASSWORd”;
private $database = “DATABASE”;
/*
Get an instance of the DBConnection
@return Instance
*/
public static function getInstance():DBConnection {
if(self::$instance==null) { // If no instance then make one
$instance=self::$instance = new DBConnection ();
}
return $instance;
}
// Constructor
private function __construct() {
$this->connection = new mysqli($this->host, $this->username,
$this->password, $this->database);
}
}
?>
Leave a Comment